Let’s briefly look at how you can create a hangman game in Javascript. We will build it step by step, explaining every single step so you will have a good understanding of how it’s working and how each piece fits into the machine, ready? Let’s begin!
Final code at the bottom!
Steps to build a hangman in Javascript
- First, create an array of words that the user can guess.
- Choose a random word from the array and store it in a variable.
- Create an array of underscores that is the same length as the chosen word.
- Create a loop that runs for as many chances as the user has to guess the word. Add a message if chances are equal to 0
- Inside the loop, get the user’s guess and check if it is in the chosen word. If it is, update the array of underscores to show the correctly guessed letter. If it is not, subtract one from the user’s chances. if all letters have been guessed inform the user of the victory.
1. Create an array of words
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
2. Choose a random word from the array
We will achieve this by using Math.random with Math.floor.
Math.random returns floating-point, a pseudo-random number that’s greater than or equal to 0 and less than 1. Math.floor returns the largest integer less than or equal to a given number.
So .random gives a random number between 0 and 0.999…, We will multiply that with our array length and use .floor on the result.
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
3. Create an array of underscores
Let’s look at two options on how to do this
1. for loop + push way
we will initiate an empty array and create for loop that will push an underscore for each letter.
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
2. .fill method
We can initiate an array and fill it up with underscores on one line. In my opinion much better and cleaner option.
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
// Set up the array of underscores
const underscores = Array(word.length).fill('_');
4. specify the number of chances and create a game loop
we will create an int with chances we want to player to give (in my case 5) and a while loop that will be active as long as chances are not 0. In that case, we will create an if statement that will tell the user that he lost.
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
// Set up the array of underscores
const underscores = Array(word.length).fill('_');
// Set the number of chances the user has to guess the word
let chances = 5;
// Run the game loop
while (chances > 0) {
}
// If the user has run out of chances, display the correct word
if (chances === 0) {
console.log(`The correct word was ${word}`);
}
5. Create the hangman game mechanic
In order to create a hangman “game” in Javascript we need to have a gameplay loop. This game will be running inside the terminal. So we need a way to get the user input. For that, we will use a library for node. You can check if you have it by running node -v. If you don’t you need to install it.
Now run the following command in your terminal to install the package:
npm install prompt-sync
after installation, we will need to paste it on top of our file
// prompt functionality to be able to play in terminal
const prompt = require("prompt-sync")({ sigint: true });
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
// Set up the array of underscores
const underscores = Array(word.length).fill('_');
// Set the number of chances the user has to guess the word
let chances = 5;
// Run the game loop
while (chances > 0) {
}
// If the user has run out of chances, display the correct word
if (chances === 0) {
console.log(`The correct word was ${word}`);
}
Lastly, focus on the while loop
1. Get users input
let’s get users to guess by using the prompt:
// prompt functionality to be able to play in terminal
const prompt = require("prompt-sync")({ sigint: true });
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
// Set up the array of underscores
const underscores = Array(word.length).fill('_');
// Set the number of chances the user has to guess the word
let chances = 5;
// Run the game loop
while (chances > 0) {
// Get the user's guess
const guess = prompt('Guess a letter: ');
}
// If the user has run out of chances, display the correct word
if (chances === 0) {
console.log(`The correct word was ${word}`);
}
2. Check if letter is guessed
Now, we need to check if a letter is in the word. we will create a boolean and a for loop that checks each letter of the word and if equals to guess, if it is then it will replace it with the letter in the underscores array. If the boolean is false then we will subtract from chances.
// prompt functionality to be able to play in terminal
const prompt = require("prompt-sync")({ sigint: true });
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
// Set up the array of underscores
const underscores = Array(word.length).fill('_');
// Set the number of chances the user has to guess the word
let chances = 5;
// Run the game loop
while (chances > 0) {
// Get the user's guess
const guess = prompt('Guess a letter: ');
// Check if the guess is in the word
let found = false;
for (let i = 0; i < word.length; i++) {
if (word[i] === guess) {
underscores[i] = guess;
found = true;
}
}
// If the guess was not found, subtract one from the chances
if (!found) {
chances--;
}
}
// If the user has run out of chances, display the correct word
if (chances === 0) {
console.log(`The correct word was ${word}`);
}
4. Show that the hangman game is actually running
At this point, I tested it and realized that we are not showing the word/underscores. I would fix it and add it sooner but I want to show that sometimes you forget about some functionality even if it’s a big one. No biggie, let’s add it!
// prompt functionality to be able to play in terminal
const prompt = require("prompt-sync")({ sigint: true });
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
// Set up the array of underscores
const underscores = Array(word.length).fill('_');
// Set the number of chances the user has to guess the word
let chances = 5;
// Run the game loop
while (chances > 0) {
// Get the user's guess
const guess = prompt('Guess a letter: ');
// Check if the guess is in the word
let found = false;
for (let i = 0; i < word.length; i++) {
if (word[i] === guess) {
underscores[i] = guess;
found = true;
}
}
// If the guess was not found, subtract one from the chances
if (!found) {
chances--;
}
// mapping each char in the string array and styling it to look like "_ _ z z "
console.log(`${underscores.map(u => u === '_' ? ' _ ' : ` ${u} `).join('')}`)
}
// If the user has run out of chances, display the correct word
if (chances === 0) {
console.log(`The correct word was ${word}`);
}
The last step is to check if the user has won. We will join the array of strings and compare them to the original word.
HANGMAN IN JAVASCRIPT FINAL CODE:
// prompt functionality to be able to play in terminal
const prompt = require("prompt-sync")({ sigint: true });
// Set up the words array
const words = ['javascript', 'programming', 'webionista', 'pizza', 'linux'];
// Select word at random
const random = Math.floor(Math.random() * words.length);
const word = words[randomNumber];
// Set up the array of underscores
const underscores = [];
for (let i = 0; i < word.length; i++) {
underscores.push('_');
}
// Set up the array of underscores
const underscores = Array(word.length).fill('_');
// Set the number of chances the user has to guess the word
let chances = 5;
// Run the game loop
while (chances > 0) {
// Get the user's guess
const guess = prompt('Guess a letter: ');
// Check if the guess is in the word
let found = false;
for (let i = 0; i < word.length; i++) {
if (word[i] === guess) {
underscores[i] = guess;
found = true;
}
}
// If the guess was not found, subtract one from the chances
if (!found) {
chances--;
}
// mapping each char in the string array and styling it to look like "_ _ z z "
console.log(`${underscores.map(u => u === '_' ? ' _ ' : ` ${u} `).join('')}`)
// Check if the user has won
if (underscores.join('') === word) {
console.log(`You won! The word was ${word}`);
break;
}
}
// If the user has run out of chances, display the correct word
if (chances === 0) {
console.log(`The correct word was ${word}`);
}
I hope I managed to teach you something here and that you found this useful. At least I hope you know How to create a hangman in Javascript. Thank you for reading.
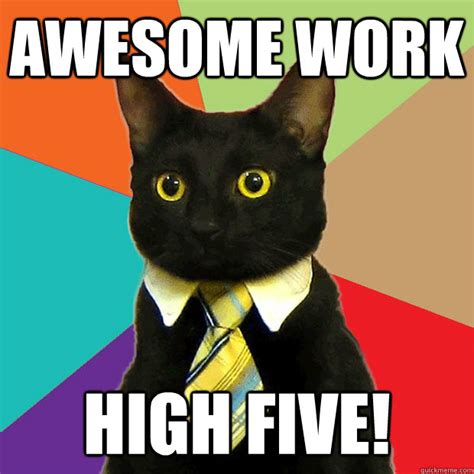